Aman Flutter
SDK
Your clients have ready access to all of Aman's payment options.
Prerequisites
Aman SDK will allow your Flutter
application to:
- initiates full-screen activities to get the shipping address, method of shipping, and payment information.
- Card, reference code, and installment payment options are easily obtained from Aman.
How Flutter
SDK Plugin Looks Like
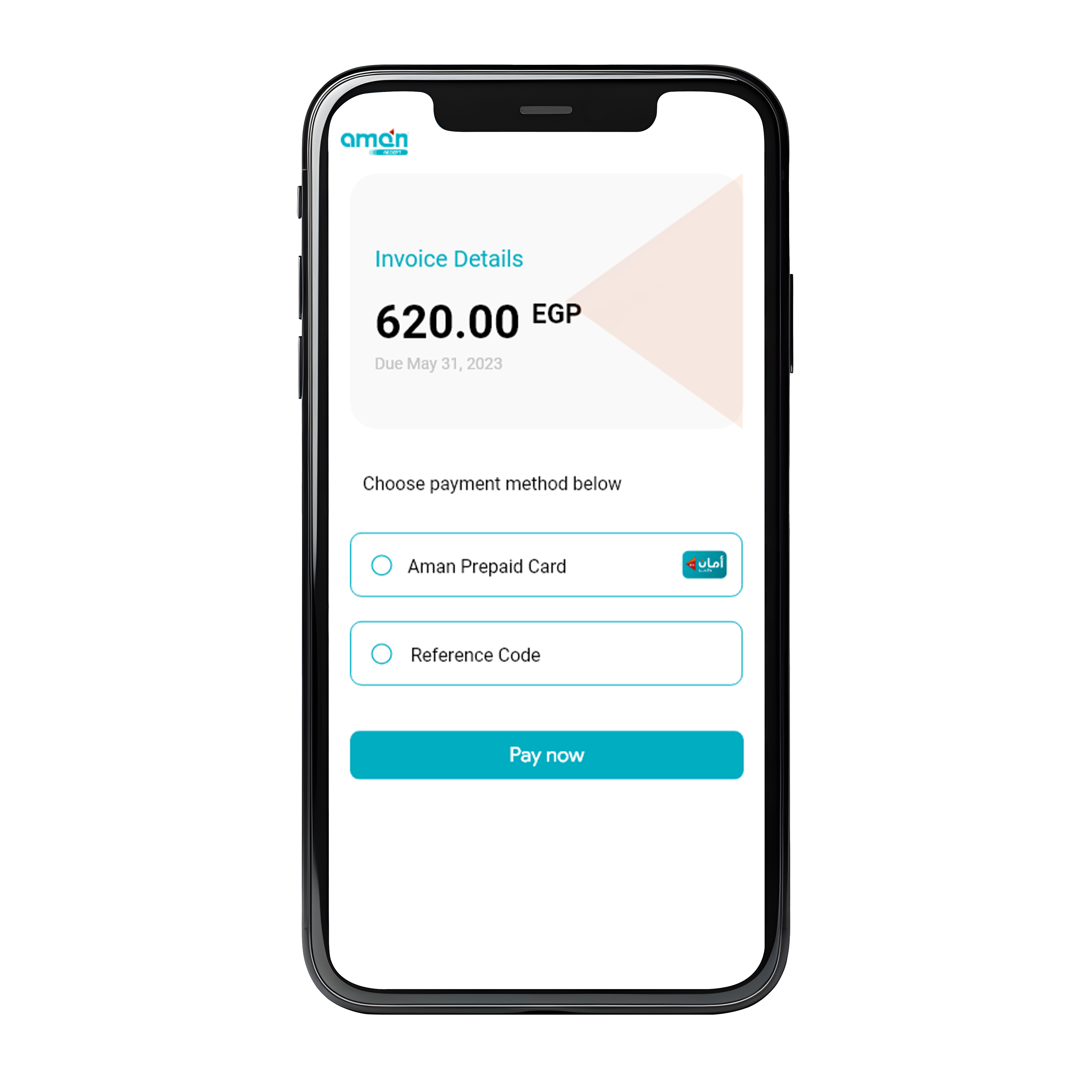

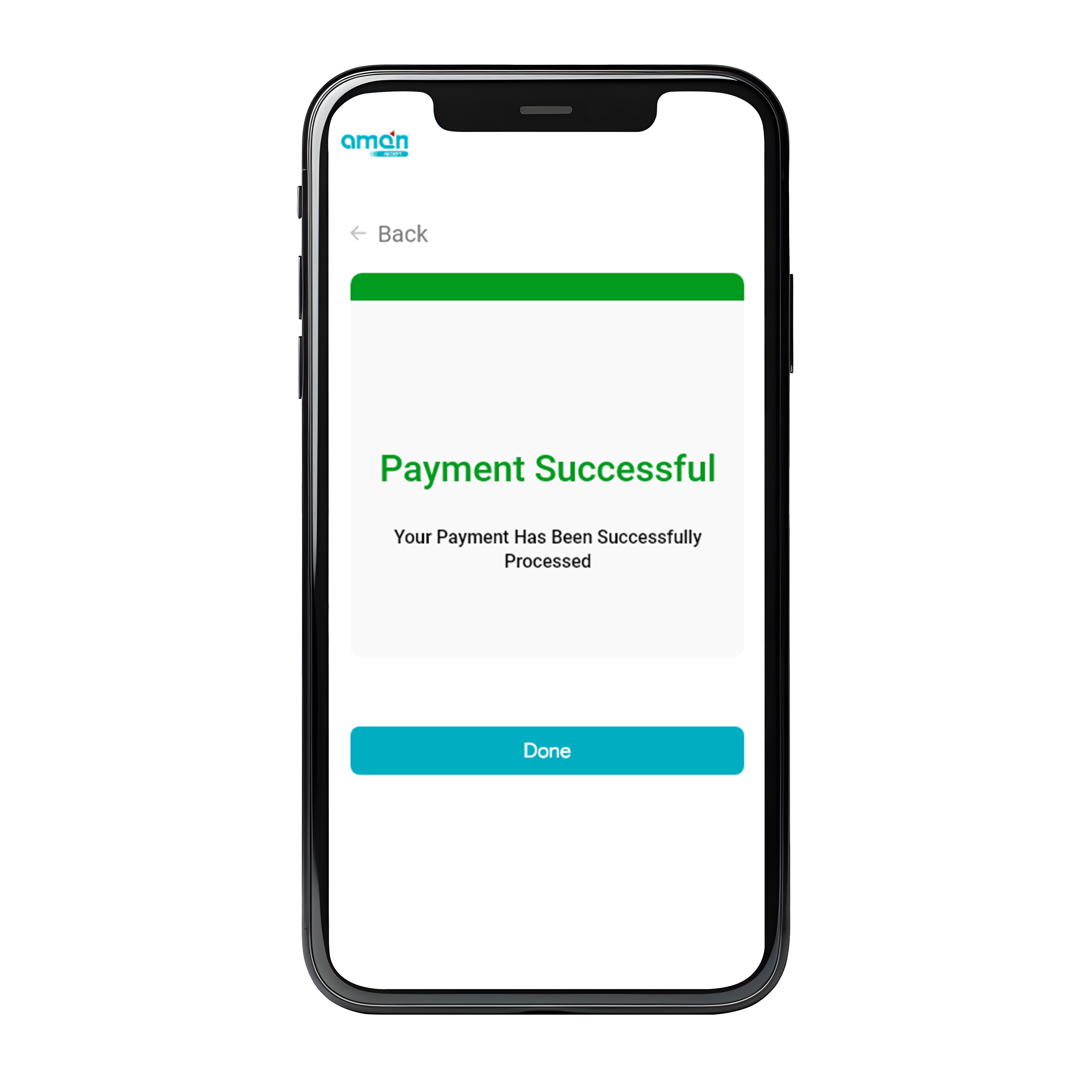
How it works
We will guide you through the Flutter
SDK integration process on
this page:
- Set up and initialize the SDK for
Flutter
. - Provide payment options and obtain the payment information from your client.
- Aman will receive a payment request from the Aman
Flutter
SDK plugin. - Give our
Flutter
SDK plugin the payment processing information back. - Share the payment outcome with your client through your
Flutter
application.
SDK Integration
To ensure that the SDK is integrated and operational within your application logic, kindly adhere to the steps listed below.
- add dependencies to your package's
pubspec.yaml
. - Run this command With
Flutter
: - Import it,
Now in your
Dart
code, you can use:
dependencies:
aman_online_flutter_sdk: "<1.0.0"
$ flutter pub get
import 'package:aman_online_flutter_sdk/aman_online_flutter_sdk.dart';
SDK API hooks
After integrating Aman SDK, now it's time to know how to use our SDK API hooks to:
- Toggle between Sandbox (testing) and Production environment.
- Create payment order.
- Handling payment result.
- Order status query.
Setup SDK Environment
You can use our SDK in testing mode while developing and integrating by configuring
the sandBox
to true:
AmanTask.setSandBox(true);
After testing is complete and your application is prepared for production, you can
toggle the sandBox
variable to false:
AmanTask.setSandBox(false);
Create Payment Order
The values that should be passed through your PayParams
object are
shown in the table below, which shows how your app cart item should implement the
PayParams
object.
Parameter | type | Required | Description | |
---|---|---|---|---|
publickey | String |
required | Your Aman merchant account public key. | |
merchantId | String |
required | Your Aman account merchant ID. | |
merchantName | String |
required | Merchant Name to be displayed in cashier checkout form. | |
reference | String |
required | Payment reference number in your system. | |
countryCode | String |
optional | Country code (EG). | |
currency | String |
required | Currency type (EGP). | |
payAmount | Long |
required | payment total amount. | |
productName | String |
required | Name of Product/Service to be purchased. | |
productDescription | String |
required | Description of Product/Service to be purchased. | |
callbackUrl | String |
required | The callback URL over which you will be listening to the payment status updates. If not configured, you have to configure a webhook url on the merchant dashboard. | |
expireAt | Integer |
required | Payment expiration time in minutes. | |
paymentType | String |
optional | The preferred payment method to be presented to your customer (BankCard, ReferenceCode, Shahry). If not set, all supported payment methods will be available to your customer to choose from. | |
userInfo JSON Object |
||||
userId | String |
optional | the customer user id | |
userName | String |
optional | the customer user name | |
userMobile | String |
optional | the customer user mobile | |
userEmail | String |
optional | the customer user email |
Create PayParams
object is given below.
var payInput = PayParams(
publicKey : "{PublicKey}",// your public key
merchantId : "256612345678901",// your merchant id
merchantName : "TEST 123",
reference : "12347544444555666",// reference unique, must be updated on each request
countryCode : "EG", // uppercase
currency : "EGP", // uppercase
payAmount : 10000,
productName : "",
productDescription :"",
callbackUrl :"http://www.callbackurl.com",
userClientIP :"110.246.160.183",
expireAt :30,
paymentType :"", // optional
//optional
userInfo = UserInfo( "userId","userEmail","userMobile","uesrName" )
)
Once the necessary PayParams
data has been initiated, you must call
AmanTask.createOrder
To begin creating an order. Here is an example of
code:
EasyLoading.show(status: "loading");
OPayTask().createOrder(context,payParams,httpFinishedMethod:(){
}).then((response){
//httpResponse (Just check the reason for the failure of the network request)
String createOrderResult=response.payHttpResponse.toJson((value){
if(value!=null){
return value.toJson();
}
return null;
}).toString();
debugPrint("httpResult=$createOrderResult");
// h5 Response (Payment result check )
if(response.webJsResponse!=null){
var status = response.webJsResponse?.orderStatus;
debugPrint("webJsResponse.status=$status");
if(status!=null){
EasyLoading.showToast(status,duration: const Duration(seconds:5));
}
switch(status){
case PayResultStatus.initial:
break;
case PayResultStatus.pending:
break;
case PayResultStatus.success:
break;
case PayResultStatus.fail:
break;
case PayResultStatus.close:
break;
}
}
});
Handling Payment Result
Your client will complete the payment, and the Aman server will reply to the SDK with the outcome of the payment processing. You will receive the payment processing result in the form of a JSON object once your client has finished paying.
Sample response Json
object:
{
"callbackName": "clickReferenceCodeReturnBtn",
"eventName": "clickReferenceCodeReturnBtn",
"merchantId": "256612345678901",
"orderNo": "211026140930379662",
"orderStatus": "PENDING", // INITIAL - SUCCESS - FAIL - CLOSE
"payNo": "211026140930379662",
"referenceCode": "607943772"
}
Order Query Status
The order status may not be updated in a timely manner due to network and other
problems. Calling order status inquiry is highly recommended if you want to make
sure your data is accurate. In order to do that, you must create an object called
CashierStatusParams
with the following request parameters:
Parameter | type | Required | Description |
---|---|---|---|
publickey | String |
required | Your Aman merchant account public key. |
merchantId | String |
required | Your Aman account merchant ID. |
reference | String |
required | Payment reference number in your system. |
countryCode | String |
required | Country code (EG). |
CashierStatusParam statusParam = CashierStatusParam(
privateKey:"OPAYPRV16204416967420.5265578503143995",
merchantId: "256621050820270",
reference:"126",
countryCode:Country.egypt.countryCode
);
After generating the CashierStatusParams
object.Use the
AmanTask().getCashierStatus(params)
method to find out the status of an
order, then proceed with the logic based on the returned response object.
EasyLoading.show(status: "loading");
OPayTask().getCashierStatus(statusParam).then((response){
EasyLoading.dismiss();
OrderInfo? data = response.payHttpResponse.data;//get result data
switch(data?.status){
case PayResultStatus.initial:
break;
case PayResultStatus.pending:
break;
case PayResultStatus.success:
break;
case PayResultStatus.fail:
break;
case PayResultStatus.close:
break;
}
setState(() {
_getCashierStatusResult=response.payHttpResponse.toJson((value){
if(value!=null){
return value.toJson();
}
}).toString();
});
});
Response data format is as follows:
{
"code": "00000",
"message": "SUCCESSFUL",
"data":{
"reference":"1001000",
"orderNo":"10212100000034000",
"status":"SUCCESS",
"vat":{
"total":10000,
"currency":"EGP",
"rate":100,
"currencySymbo":"ج.م"
},
"failureReason":null,
"silence":"Y"
}