Aman React Native
SDK
Your clients have ready access to all of Aman's payment options.
Prerequisites
Aman SDK will allow your React Native
application to:
- initiates full-screen activities to get the shipping address, method of shipping, and payment information.
- Card, reference code, and installment payment options are easily obtained from Aman.
Make sure you are satisfied with the following compatibility list in order to prevent any conflicts and ensure a smooth integration.
Item | COMPATIBLE VERSION | REFERENCES |
---|---|---|
react-native-webview |
>=8.0.0 | |
react-native |
>=0.51 | |
crypto-js |
>=3.2.0 |
How React Native
SDK Plugin Looks Like
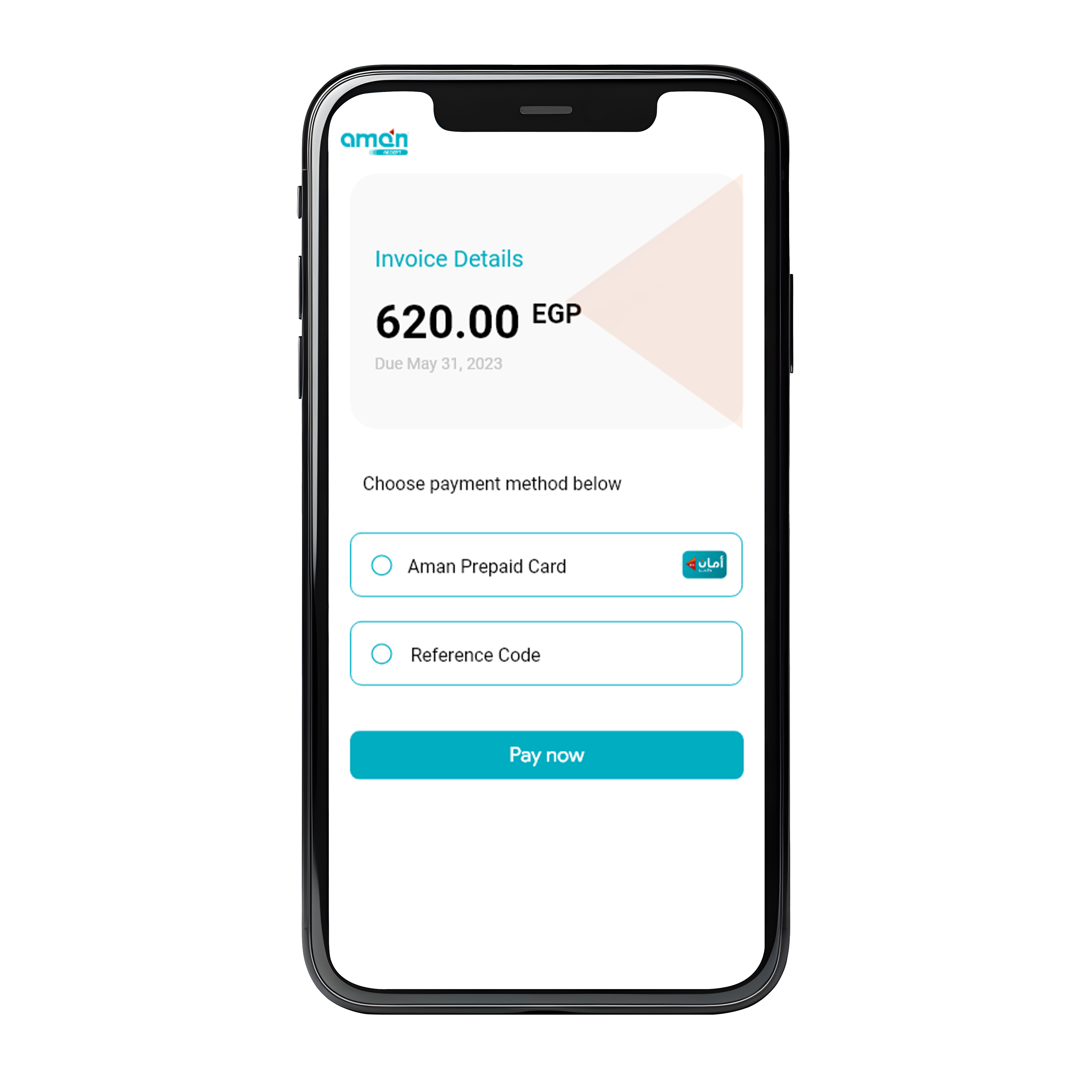

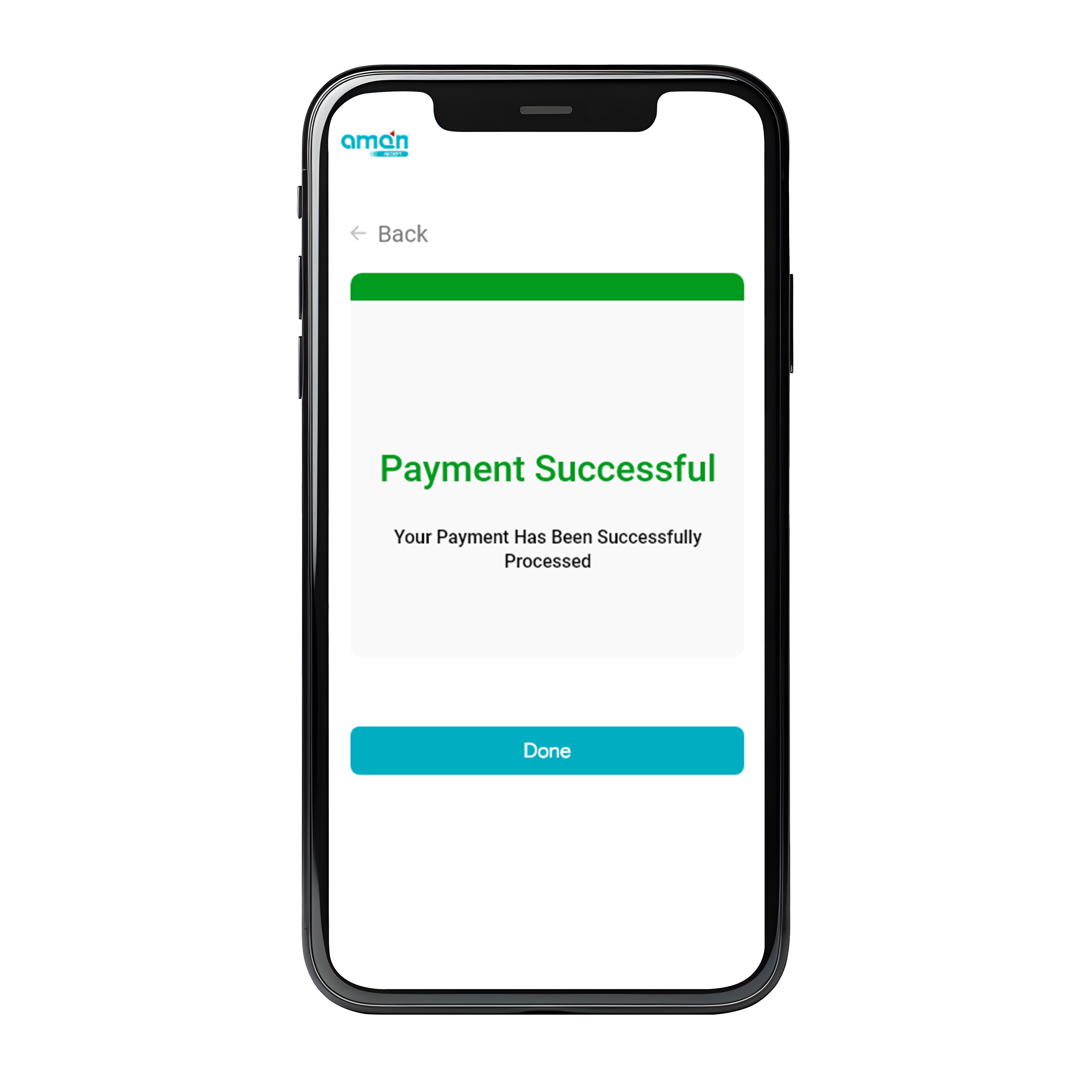
How it works
We will guide you through the React Native
SDK integration process
on this page:
- Set up and initialize the SDK for
React Native
. - Provide payment options and obtain the payment information from your client.
- Aman will receive a payment request from the Aman
React Native
SDK plugin. - Give our
React Native
SDK plugin the payment processing information back. - Share the payment outcome with your client through your
React Native
application.
SDK Integration
To ensure that the SDK is integrated and operational within your application logic, kindly adhere to the steps listed below.
- If you already have the previous version, you must delete it first. Run:.
- in your project directory,run:
- Installing dependencies into a bare React Native project:
npm uninstall aman-online-rn-sdk
yarn remove aman-online-rn-sdk
npm:
npm install aman-online-payment-rn-sdk
yarn:
yarn add aman-online-payment-rn-sdk
npm:
npm install react-native-webview
yarn:
yarn add react-native-webview
If your React Native is 0.59 and lower,you need to linking.
react-native link react-native-webview
From React Native 0.60 and higher, linking is automatic.If you're on a Mac and developing for iOS, you need to install the pods (via Cocoapods) to complete the linking.
cd ios
pod install
SDK API hooks
After integrating Aman SDK, now it's time to know how to use our SDK API hooks to:
- Toggle between Sandbox (testing) and Production environment.
- Create payment order.
- Handling payment result.
- Order status query.
Setup SDK Environment
You can use our SDK in testing mode while developing and integrating by configuring
the sandBox
to true:
Aman.isSandBox=true
After testing is complete and your application is prepared for production, you can
toggle the sandBox
variable to false:
Aman.isSandBox=false
Create Payment Order
add AmanPage(A Component)
into >react-navigation route
config.
The values that should be passed through your PayParams
object are
shown in the table below, which shows how your app cart item should implement the
PayParams
object.
Parameter | type | Required | Description | |
---|---|---|---|---|
publickey | String |
required | Your Aman merchant account public key. | |
merchantId | String |
required | Your Aman account merchant ID. | |
merchantName | String |
required | Merchant Name to be displayed in cashier checkout form. | |
reference | String |
required | Payment reference number in your system. | |
countryCode | String |
optional | Country code (EG). | |
currency | String |
required | Currency type (EGP). | |
payAmount | Long |
required | payment total amount. | |
productName | String |
required | Name of Product/Service to be purchased. | |
productDescription | String |
required | Description of Product/Service to be purchased. | |
callbackUrl | String |
required | The callback URL over which you will be listening to the payment status updates. If not configured, you have to configure a webhook url on the merchant dashboard. | |
expireAt | Integer |
required | Payment expiration time in minutes. | |
paymentType | String |
optional | The preferred payment method to be presented to your customer (BankCard, ReferenceCode, Shahry). If not set, all supported payment methods will be available to your customer to choose from. | |
userInfo JSON Object |
||||
userId | String |
optional | the customer user id | |
userName | String |
optional | the customer user name | |
userMobile | String |
optional | the customer user mobile | |
userEmail | String |
optional | the customer user email |
Create PayParams
object is given below.
let payInput = {
publicKey : "{PublicKey}",// your public key
merchantId : "256612345678901",// your merchant id
merchantName : "TEST 123",
reference : "12347544444555666",// reference unique, must be updated on each request
countryCode : "EG", // uppercase
currency : "EGP", // uppercase
payAmount : 10000,
productName : "",
productDescription :"",
callbackUrl :"http://www.callbackurl.com",
userClientIP :"110.246.160.183",
expireAt :30,
paymentType :"", // optional
// optional
userInfo = {
userId:"userId",
userEmail:"userEmail",
userMobile:"userMobile",
userName:"uesrName"
}
}
Once the necessary PayParams
data has been initiated, you must call
props.navigation.push()
to start AmanPage to begin creating an order.
Here is an example of code:
this.props.navigation.push("OPayPageRouteName", // your defined route name
{
payParams:this.payParams,
httpCallback:this.orderHttpCallback,
webPayCallback:this.orderWebPayCallback,
});
/**
* http callback from order api
* @param response
*/
orderHttpCallback=(response)=>{
console.log(JSON.stringify(response))
}
/**
* web callback from pay page than behind order api
* @param webJsResponse
*/
orderWebPayCallback=(webJsResponse:OPayWebJsResponse)=>{
if(webJsResponse!=null){
let status = webJsResponse.orderStatus;
console.log(JSON.stringify(webJsResponse))
switch(status){
case PayResultStatus.initial:
break;
case PayResultStatus.pending:
break;
case PayResultStatus.success:
break;
case PayResultStatus.fail:
break;
case PayResultStatus.close:
break;
}
}
}
Handling Payment Result
Your client will complete the payment, and the Aman server will reply to the SDK with the outcome of the payment processing. You will receive the payment processing result in the form of a JSON object once your client has finished paying.
Sample response Json
object:
{
"callbackName": "clickReferenceCodeReturnBtn",
"eventName": "clickReferenceCodeReturnBtn",
"merchantId": "256612345678901",
"orderNo": "211026140930379662",
"orderStatus": "PENDING", // INITIAL - SUCCESS - FAIL - CLOSE
"payNo": "211026140930379662",
"referenceCode": "607943772"
}
Order Query Status
The order status may not be updated in a timely manner due to network and other
problems. Calling order status inquiry is highly recommended if you want to make
sure your data is accurate. In order to do that, you must create an object called
CashierStatusParams
with the following request parameters:
Parameter | type | Required | Description |
---|---|---|---|
publickey | String |
required | Your Aman merchant account public key. |
merchantId | String |
required | Your Aman account merchant ID. |
reference | String |
required | Payment reference number in your system. |
countryCode | String |
required | Country code (EG). |
let cashierStatusParams ={
privateKey:"OPAYPRV16387746262010.7102038091157474",
merchantId:"281821120675251",
reference:"123",
countryCode : OPayCountry.EGYPT.countryCode
}
After generating the CashierStatusParams
object.Use the new
Aman().getCashierStatus(params)
method to find out the status of an
order, then proceed with the logic based on the returned response object.
new Aman().getCashierStatus(cashierStatusParam).then((response){
let status = response.data.status
switch(status){
case PayResultStatus.initial:
break;
case PayResultStatus.pending:
break;
case PayResultStatus.success:
break;
case PayResultStatus.fail:
break;
case PayResultStatus.close:
break;
}});
Response data format is as follows:
{
"code": "00000",
"message": "SUCCESSFUL",
"data":{
"reference":"1001000",
"orderNo":"10212100000034000",
"status":"SUCCESS",
"vat":{
"total":10000,
"currency":"EGP",
"rate":100,
"currencySymbo":"ج.م"
},
"failureReason":null,
"silence":"Y"
}